Adding XAPP to your Project
Using Cocoapods
Add the pod to the desired target:
pod 'XAPP'
Alternate methods
See our guide for alternate methods for integrating the SDK to your project.
Basic Usage Objective-C
In addition to these basic usage instructions, please see the example code.
Starting the Session
In your primary app delegate import XAPP.h alongside the other header imports.
Setup Credentials in your Info.plist
Your credentials need to be in your app’s plist in order for the SDK to read them.
<key>XAPP</key>
<dict>
<key>apiKey</key>
<string>your-api-key</string>
<key>appKey</key>
<string>your-app-key</string>
</dict>
See the full implementation in this example.
You can use the supplied xapp.sh
script to set it for you, which you can run once or as a build phase script.
Go to your target’s Build Phases, then add a new Run Script
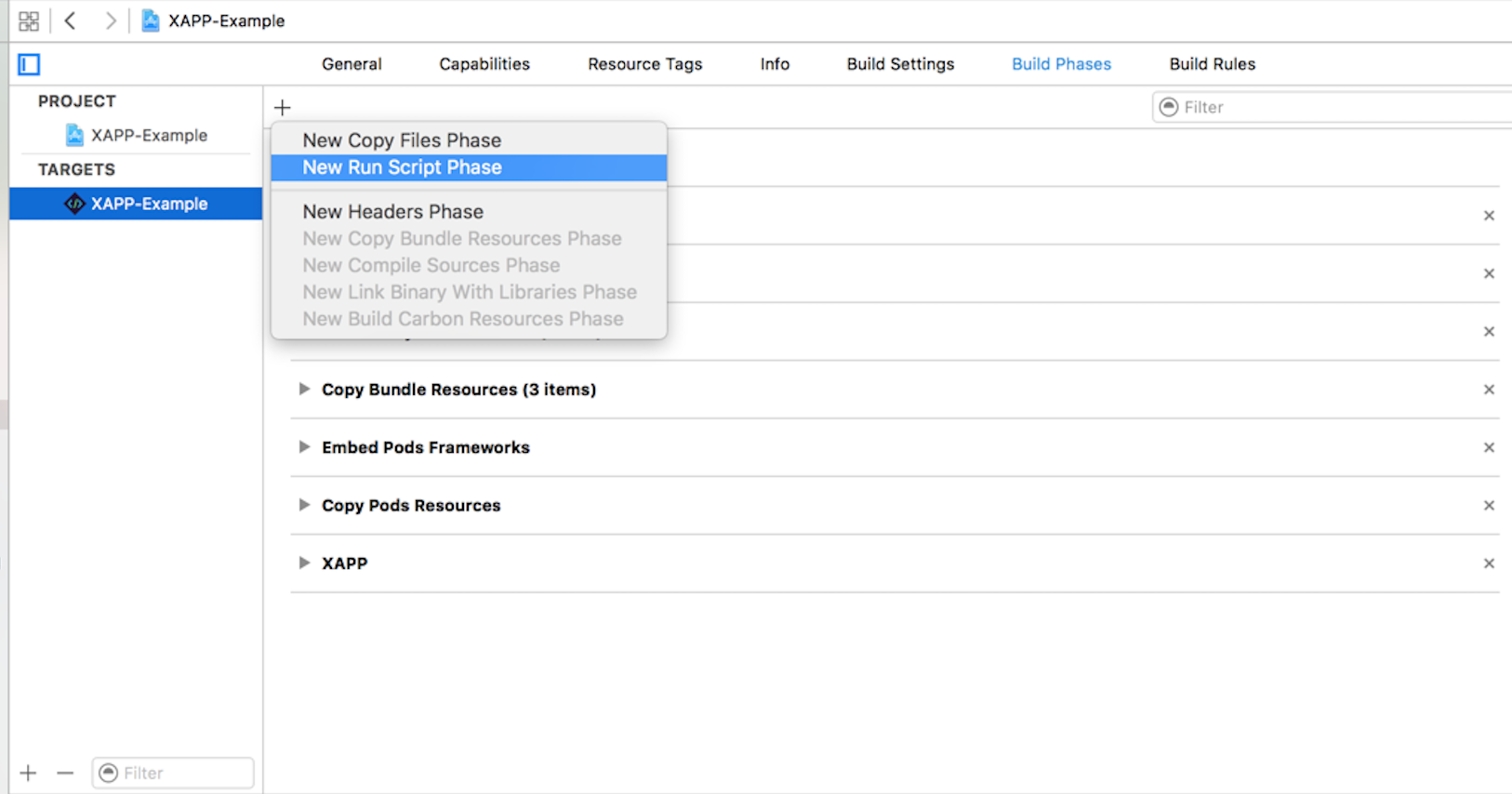
Copy and paste the following script:
Pods/XAPP/XAPP.framework/xapp.sh -api=<% API Key %> -app=<% APP Key %>
Note Pod/XAPP/XAPP.framework
is a variable path, this path will change depending where you import the XAPP.framework.
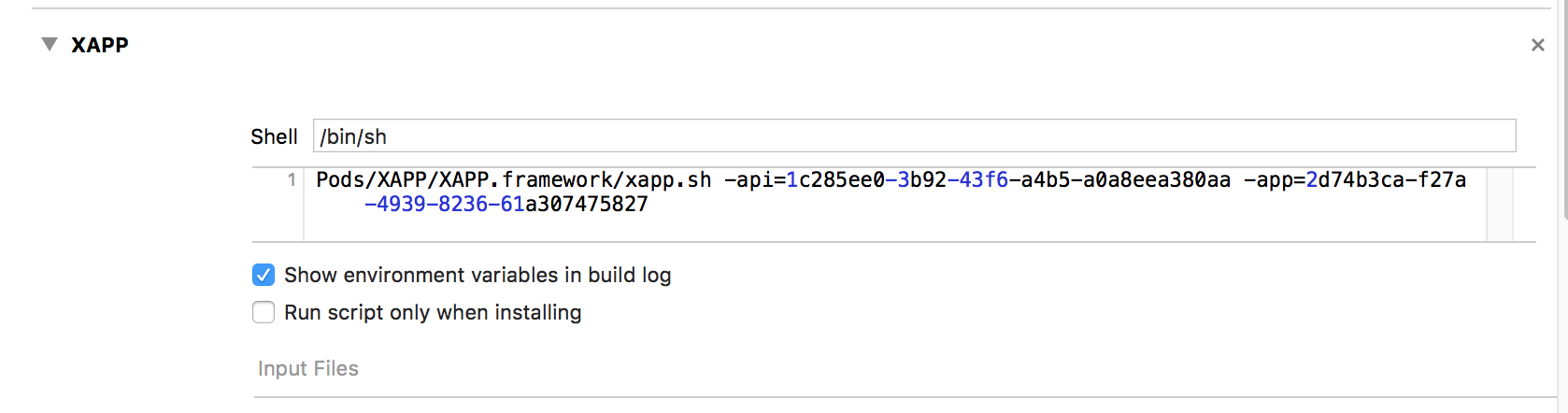
Now, in your app delegate, call [XAPP startSession]
:
#import <XAPP/XAPP.h>
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch.
[XAPP startSession];
return YES;
}
An alternative to storing your credentials in your plist is to use string constants with [XAPP startSessionWithAPIKey:withApplicationKey]
Requesting a XAPP
Depending on if your existing ad request workflow, there are two primary ways to request a XAPP.
If you:
- Already have a ad server have a workflow to get ad metadata to your app
- Want to request a known specific XAPP
//Make sure you have XAPP imported
#import <XAPP/XAPP.h>
//hold reference for advertisement object
@property (weak, nonatomic) XAAdvertisement *ad;
XARequest *tagRequest = [XARequest requestWithTag:@"tag"];
tagRequest.userData = self.userData; //pass userdata
[XAPP requestAd:request success:^(XAAdvertisement *advertisement) {
NSLog(@"Request returned %@", advertisement.adTitle);
self.ad = advertisement;
} failure:^(NSError *error) {
NSLog(@"Request failed %@", error ? [NSString stringWithFormat:@"with error: %@", error.localizedDescription] : @"");
}];
Otherwise, if you:
- Already have a DFP ad server and want to use the SDK to request an ad
- Want to leverage XAPP for ad serving
Use [XAPP adRequestForDfpWithNetworkId:andAdUnit:withParameters:]
Playback
Play the advertisement as an interstitial, where the companion image takes up the entire screen:
//The rootViewController is a reference to the UIViewController you want the ad to display in
[XAPP playAdAsInterstitial:self.ad withRootViewController:self startedPlayback:^(XAAdvertisement *advertisement) {
} success:^(XAAdvertisement *spot, XAResult *result) {
} failure:^(XAAdvertisement *spot, NSError *error) {
}];
Or play the ad with the companion image inside a confined tile:
//The containing view is the view for the advertisement to display within. It must inherit from UIView.
[XAPP playAdAsInTunerTile:self.ad withContainingView:self.inTunerTileView startedPlayback:^(XAAdvertisement *advertisement) {
} success:^(XAAdvertisement *spot, XAResult *result) {
} failure:^(XAAdvertisement *spot, NSError *error) {
}];
Or play the ad as audio only, without a companion image:
[XAPP playAdAsAudioOnly:self.ad startedPlayback:^(XAAdvertisement *advertisement) {
} success:^(XAAdvertisement *spot, XAResult *result) {
} failure:^(XAAdvertisement *spot, NSError *error) {
}];
Instead of storing the advertisement locally, you can retrieve the next advertisement from XAPP through:
if ([XAPP adAvailable]) {
XAAdvertisement * advertisement = [XAPP nextAd];
[XAPP playAdAsInTunerTile:advertisement withContainingView:tileImageView
startedPlayback:^(XAAdvertisement *advertisement) {
} success:^(XAAdvertisement *advertisement, XAAdResult *result) {
} failure:^(XAAdvertisement *advertisement, NSError *error) {
}];
}
Basic Usage Swift
In addition to these basic usage instructions, please see the example code.
Import Headers
In your bridge header import XAPP.h alongside the other imports:
#import <XAPP/XAPP.h>
#import <XAPP/XAAction.h>
#import <XAPP/XARequest.h>
Start the Session
let apiKey = "<#API Key#>"
let appKey = "<#Application Key#>"
var adView = XAAdvertisement.init(nil);
XAPP.startSessionWithAPIKey(apiKey, withApplicationKey: appKey)
After starting XAPP with your apiKey and appKey, request an Advertisement:
let request = XARequest()
request.tag = "AD TAG"
XAPP.requestAd(request, success: { (spot) in
self.adView = spot
}) { (error) in
}
Display the Advertisement, display as an interstitial, which takes up the entire screen:
XAPP.playAdAsInterstitial(self.adView, withRootViewController: self, startedPlayback: { (adver) in
}, success: { (adver, res) in
}) { (adver, err) in
self.requestAdButton.enabled = true
}
Or display the ad inside a confined tile:
XAPP.playAdAsInTunerTile(self.adView, withContainingView: self.inTunerTileView, startedPlayback: { (adver) in
}, success: { (adver, res) in
}) { (adver, err) in
self.requestAdButton.enabled = true
}
Or play the ad as audio only:
XAPP.playAdAsAudioOnly(self.adView, startedPlayback: { (adver) in
}, success: { (adver, res) in
}) { (adver, err) in
self.requestAdButton.enabled = true
}
Time to Test
Complete your integration by running through our recommended test cases.