Intro
XAPP SDK comes with a default microphone animation. As in following gif.
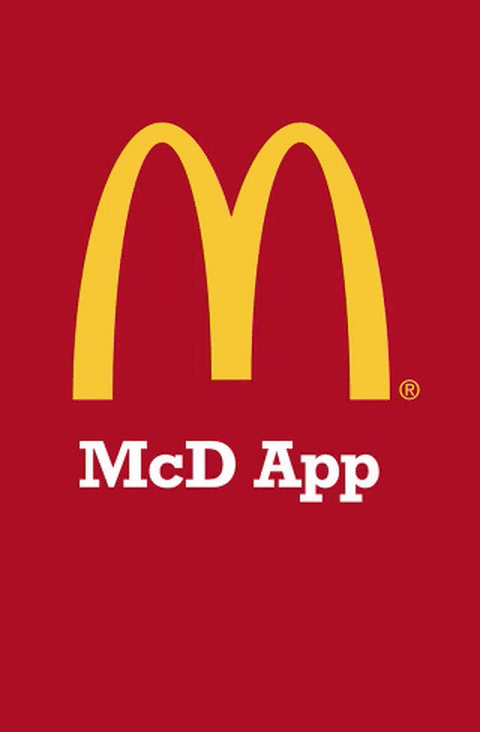
When advertisement is presented as interstitial or in-tuner tile , XAPP SDK adds a blur view to image frame then displays the default mic animations on the bottom of the image frame.
Using Default Mic Animation
To use default animation you can use following code before you make an adRequest
[XAPP microphoneAnimation:nil displayAnimation:YES];
or to disable microphone animations completely you can use
[XAPP microphoneAnimation:nil displayAnimation:NO];
Custom Mic Animations
You have the option of overriding the default animation with your own custom animation.
All you need to do is to adapt XAMicAnimationProtocol
protocol in your UIView
or UIViewController
class
#import <XAPP/XAMicAnimationProtocol.h>
@interface XMMicAnimationCircular : UIView<XAMicAnimationProtocol>
Once your class is implemented the protocol methods , you can initiate your class with desired frame size and pass your class to XAPP SDK.
const CGFloat circular = 100.0;
CGPoint center = CGPointMake(CGRectGetMidX(self.view.bounds), CGRectGetMidY(self.view.bounds));
XMMicAnimationCircular *animationView = [[XMMicAnimationCircular alloc] initWithFrame:CGRectMake(center.x-circular, center.y-circular,circular*2, circular*2)];
[animationView updateFrame:CGRectMake(center.x-circular, center.y-circular,circular*2, circular*2)];
[XAPP microphoneAnimation:animationView displayAnimation:YES];
How XAPP SDK is Using Your Custom Mic Animation.
XAPP SDK checks if your custom animation class belongs one of the UIView
or UIViewController
classes, if so it adds your class to advertisement view.
Please make sure you either use either UIView
or UIViewController
as your class of choice, unfortunately XAPP SDK doesn’t work directly with Core Animation Layers
at the moment.
Adding animation to your view is in your responsibility.
setHidden
After adding animation view to advertisement view, XAPP SDK calls
- (void)setHidden:(BOOL)hidden;
method of your class to hide or display animation during the speech listening state.
## updateFrame
XAPP SDK sometimes reset layouts for the advertisement before displaying the advertisement view.
Ideally calling this method should reset the frame of your custom view to given ratio by XAPP.
For example your updateFrame function may look like this this;
- (void)updateFrame:(CGRect)frame {
self.frame = frame;
}
## startAnimatingWithLevel
When xapp starts listening for speech, this method sends decibel levels of users voice as float values from 0.000 to 1.000 roughly about every 150 ms for about 2 seconds.
After two seconds your custom animation will be either hidden or will be removed from view.
An example of implementing this method in your custom animation class
- (void)startAnimatingWithLevel:(CGFloat)level
{
//set a boolean to check if animation started because this method will be called by xapp everytime decibel levels are available
if (!self.animate) {
//maybe your start animation function
[self startAnimating];
}
//do whatever you like with the float value to make animation pretty
}
## stopAndRemoveLayer
This method will be called by XAPP SDK when voice recognition is done and SDK is ready to remove the animation from the view.
# Example Animations
You can clone or download this project to explore more animations or to test your own animations.
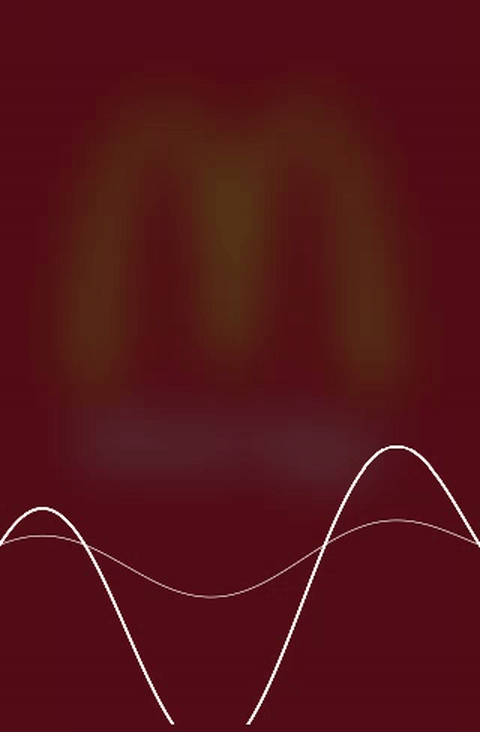
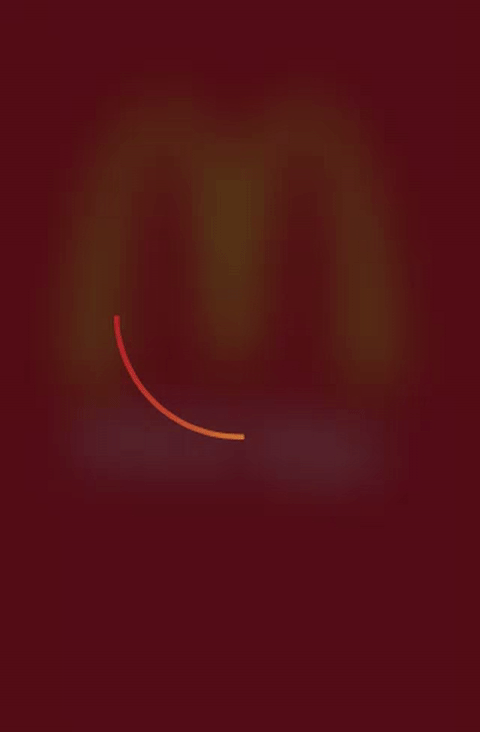
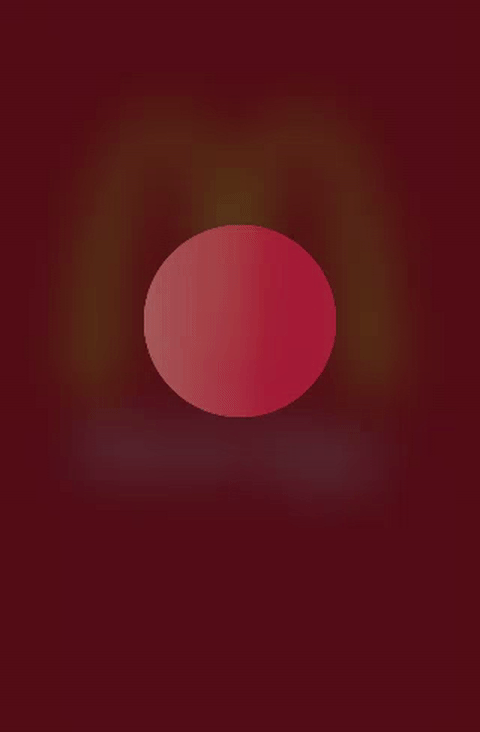
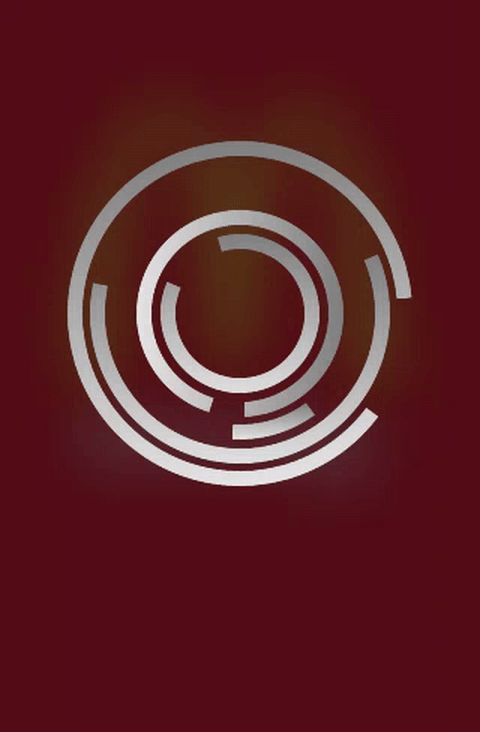