Overview
Version 4.0 of the iOS XAPPmedia SDK was built on the idea to make the SDK easier to implement by removing or automating a lot of what it took to get from start to finish. As a result, the public API took quite a big overhaul. The goal of this guide is to ease the transition from 3.x to 4.x.
Using Cocoapods
Original Pod name ‘XappAds’ has been changed to ‘XAPP’ in 4.0, please update your podfile and use the ‘XAPP’ pod:
pod 'XAPP'
Headers
Prefix has been changed from XappAds to XAPP in order to access the headers you need to replace
#import <XappAds/
with #import <XAPP/
For example in order to access the main facade you need to add #import <XAPP/XAPP.h>
in your app.
Also prefix for the other headers are edited. Ad
prefix has been removed from all headers.
For example XAAdRequest.h
is now XARequest.h
Removed Headers
The deferred manager is not longer needed, we folded the usage into [XAPP startSession]
.
#import <XappAds/XADeferredManager.h>
We also removed the delegate in favor of blocks.
#import <XappAds/XappAdsDelegate.h>
The once deprecated XADFPAdapter
has finally been removed
#import <XappAds/XADFPAdapter.h>
and the seldom used XADiagnosticEvents
class is gone.
#import <XappAds/XADiagnosticEvents.h>
Modified Headers
We replaced the default microphone animation and implemented a protocol for custom microphone animations.
#import <XappAds/XKMeterView.h>
If you want to use a custom microphone animation use #import <XAPP/XAMicAnimationProtocol.h>
, see the Mic Animations Guide for more information.
We no longer use an XAAdView
as the reference for the advertisement, we instead expose the underlying XAAdvertisement object.
Remove
#import <XappAds/XAAdView.h>
and instead import
#import <XAPP/XAAdvertisement.h>
Session Start
The SDK is authorized by placing your credentials within your app’s Info.plist
. You can use the supplied xapp.sh
script to set it for you, which you can run once or as a build phase script.
Go to your target’s Build Phases, then add a new Run Script
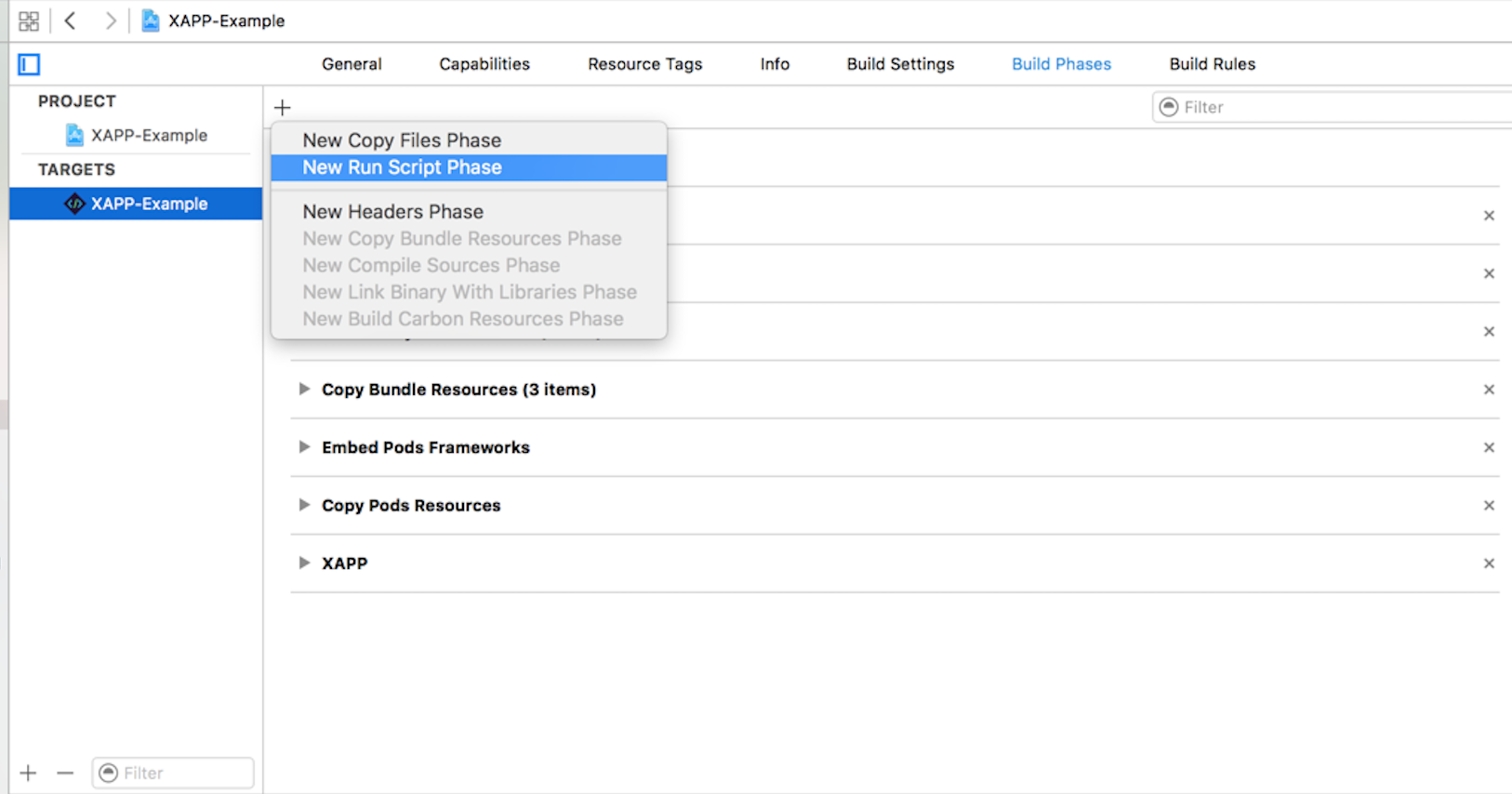
Copy and paste the following script:
Pods/XAPP/XAPP.framework/xapp.sh -api=<% API Key %> -app=<% APP Key %>
Then change the session start code from:
- (void)viewDidLoad {
//Start XappAds
[XappAds startWithAPIKey:myAPIKey
withApplicationKey:myApplicationKey
withAdsDelegate:self
withUserData:nil];
}
- (void)onXappAdsStarted {
//now request an ad
}
to:
//Within your application delegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch.
//Start the session, no need to listen for a callback.
[XAPP startSession];
return YES;
}
Request XAPP
Requests are changed from:
XAAdRequest *request = [XAAdRequest adRequestWithAdName:@"name"];
[Xappads requestAd:request withResponse:^(BOOL success, XAAdRequest *request, XAAdvertisement *advertisement, NSArray *metadata, NSError *error) { }];
to (also pass the user data to request)
XARequest *request = [XARequest requestWithTag:@"tag"];
request.userData = self.userData; //pass userdata, optional
[XAPP requestAd:request success:^(XAAdvertisement *spot) {
//use the XAAdvertisement obj to play the ad
self.receivedAd = spot;
} failure:^(NSError *error) {
//do something with the error
}];
Note: Make sure you have the correct variables to keep the xapp and send the user data In your @interface` add something like following
//Private
@property (weak, nonatomic) XAAdvertisement *receivedAd;
@property (retain, nonatomic) XAUserData *userData;
Play XAPP
Playing ads went from:
- (void)play {
[XappAds playAdAsInterstitial:adView withRootViewController:self];
}
//with delegate method
- (void)adFinishedPlayBack:(XAAdView *)adView withResult:(XAAdResult *)result andError:(NSError *)error {
NSLog(@"Ad finished playback");
}
to:
[XAPP playAdAsInterstitial:self.receivedAd withRootViewController:self.tabBarController
startedPlayback:^(XAAdvertisement *advertisement) {
} success:^(XAAdvertisement *advertisement, XAResult *result) {
} failure:^(XAAdvertisement *advertisement, NSError *error) {
}];
For in-tuner tile and audio only ads simply call the playAdAsInTunerTile
or playAdAsAudioOnly
methods, for example:
[XAPP playAdAsAudioOnly:self.receivedAd startedPlayback:^(XAAdvertisement *advertisement) {
} success:^(XAAdvertisement *advertisement, XAResult *result) {
} failure:^(XAAdvertisement *advertisement, NSError *error) {
}];
[XAPP playAdAsInTunerTile:self.receivedAd withContainingView:viewController.tileView
startedPlayback:^(XAAdvertisement *advertisement) {
} success:^(XAAdvertisement *advertisement, XAResult *result) {
} failure:^(XAAdvertisement *advertisement, NSError *error) {
}];